The Setup
We need to start with the required tools to getting the application setup.
IDE Enviroment
Firstly we are going to need an IDE, you can use any IDE such as Netbeans or Eclipse.
For this demo I will be using eclipse. Click this link for help with installing Eclipse. Don't create any projects, just get to the step before you create a project. Ensure you install the Java EE Edition (This is a Java IDE more catered for web development).
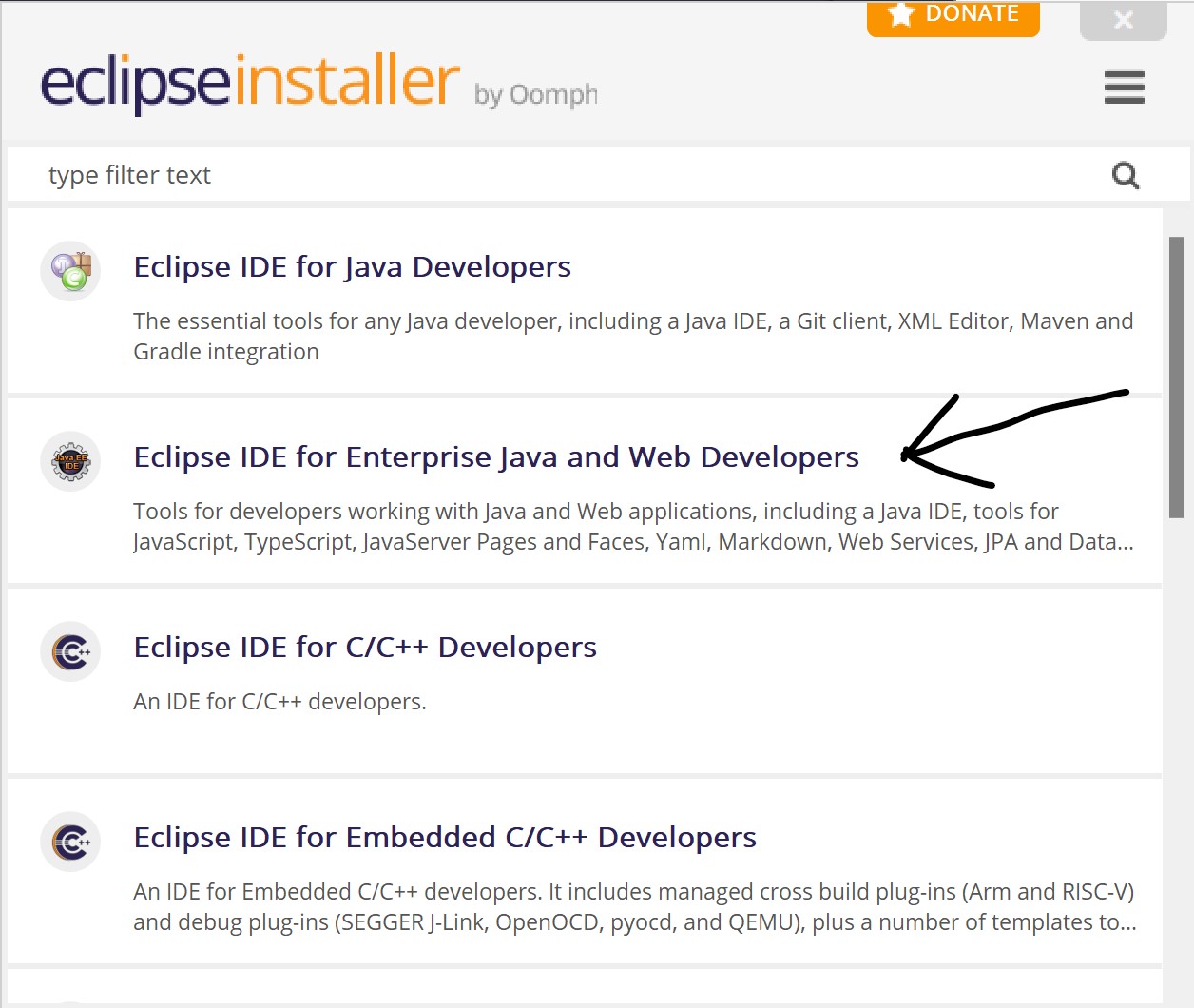
Database Enviroment
Secondly we will need a way to persit the data. For this demo we will use MySQL as the database.
Click this for help with installing MySQL. Use the Developer Default Settings.
Once you have completed the installation, download this script file and run it inside the MySQL Workbench application with your root login.
It will create a user for the MySQL data, with the username StudentAdmin (password = password of course, because it's the most secure password that is known to man).
Then the script will create a few tables, which I will go into more depth later. After the tables have been created it will enter some dummy data to get us started.
MySQL is very similar to Oracle, so I won't go into the details on the differences but click here for more details on MySQL (including the differences from Oracle).
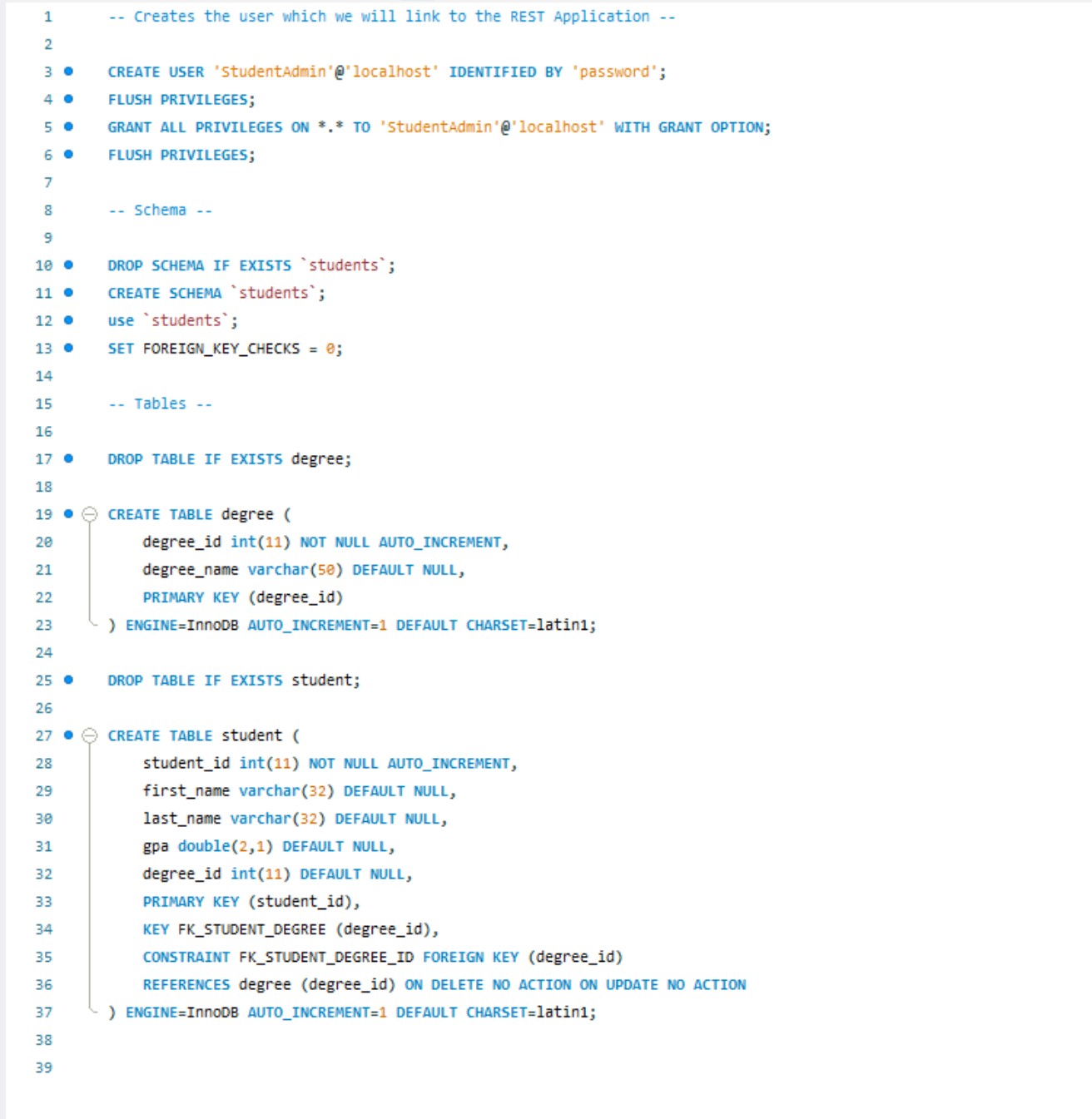
Downloading the Spring Boot Starter Project
Lastly, we will configure the project and download the project initializer files
Go to this website to configure the starter project.
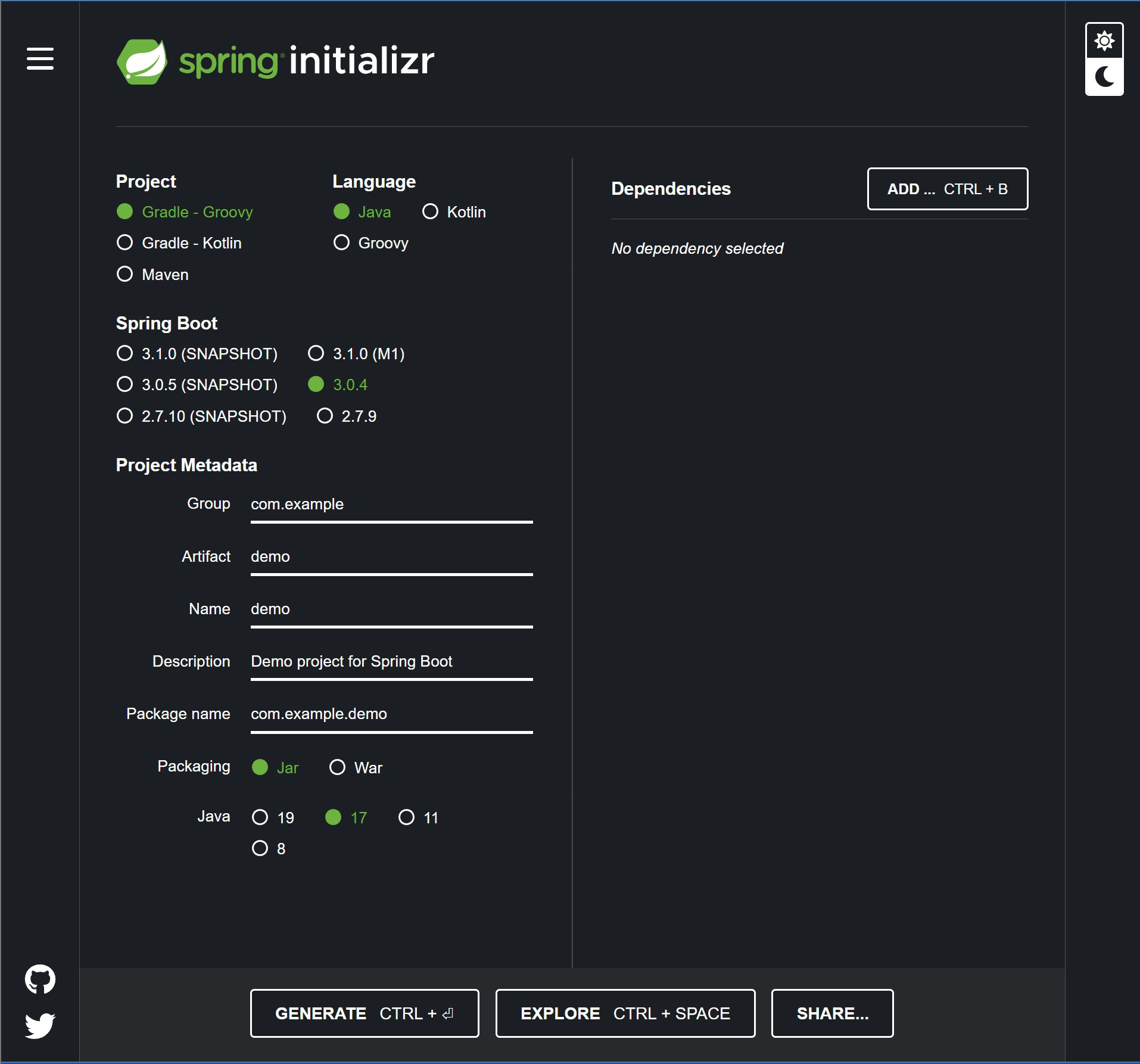
Configuration:
- Project - Maven
- Language - Java
- Spring Boot - 3.0.4 (Any Current Version that isn't SNAPSHOT will do).
- Packaging - Jar (Allows us to package the application up into a runnable Jar file).
- Java Version - 17.
-
Dependencies -
- Spring Web (Embedded webserver and REST Controller) - Required
- Spring Boot DevTools (Mainly for LiveReload and fast restarts when making changes) - Not Required
- MySQL Driver (Established the connection to the database) - Required
- Spring Data JPA (Interaction with the database) - Required
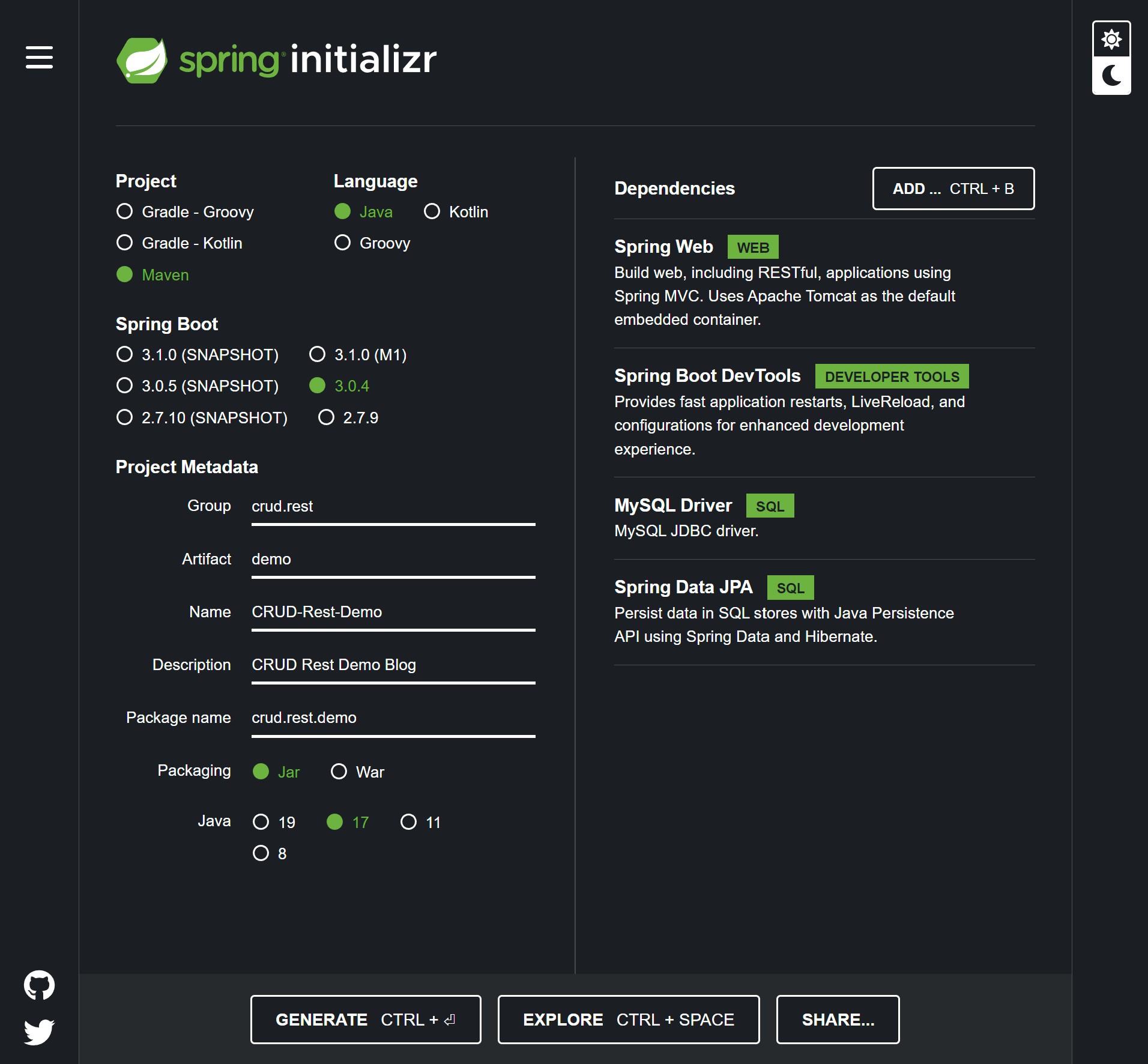
Click generate to download the initalizr project. Click here to download project if you don't want to generate your own.
Import the Maven Project
The final step for the setup is to just import the project.
Go to File > Import > Maven > Existing Maven Project.
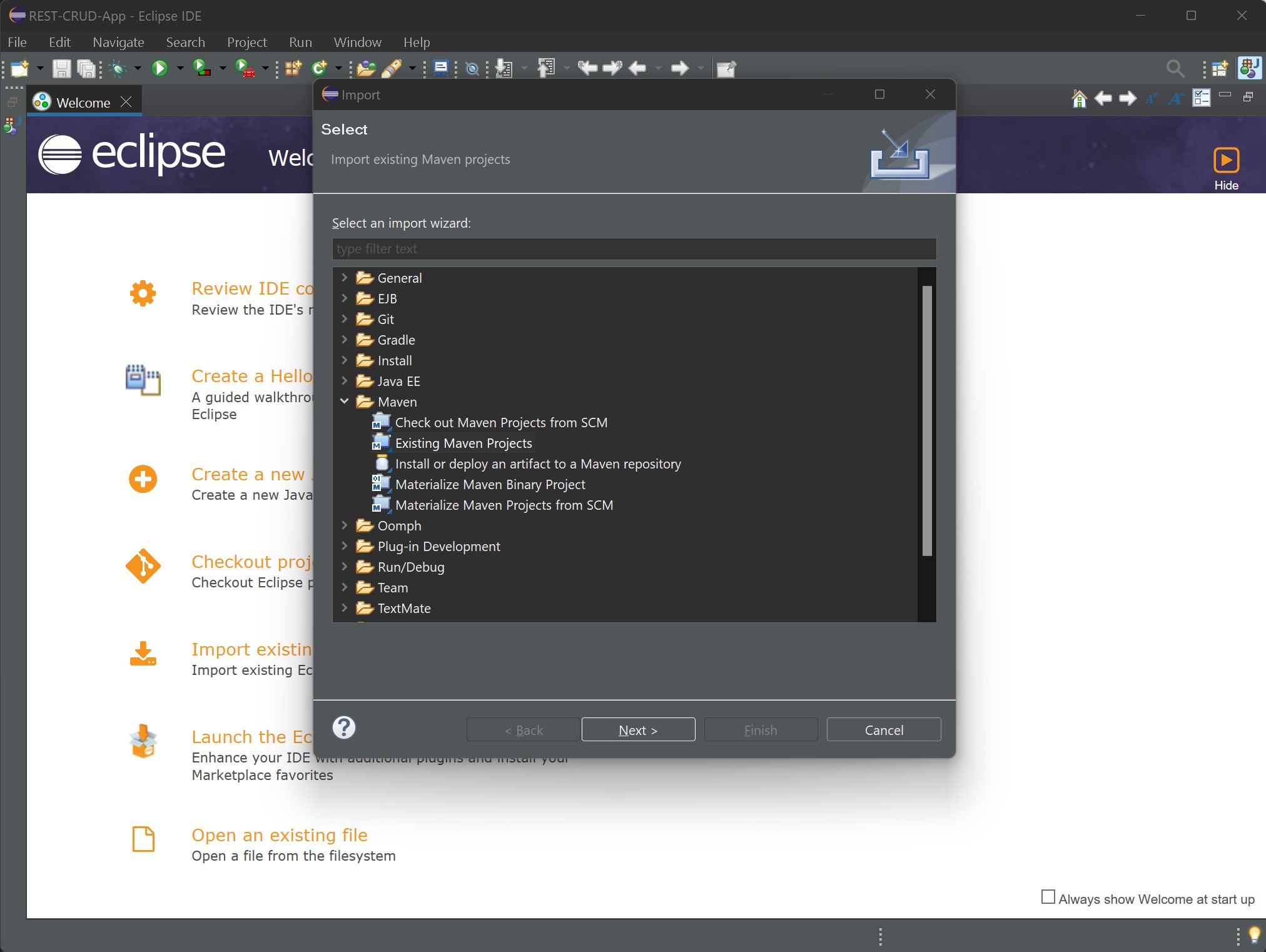
Select the location of the Maven Folder > Select the POM.xml file > Finish Button.
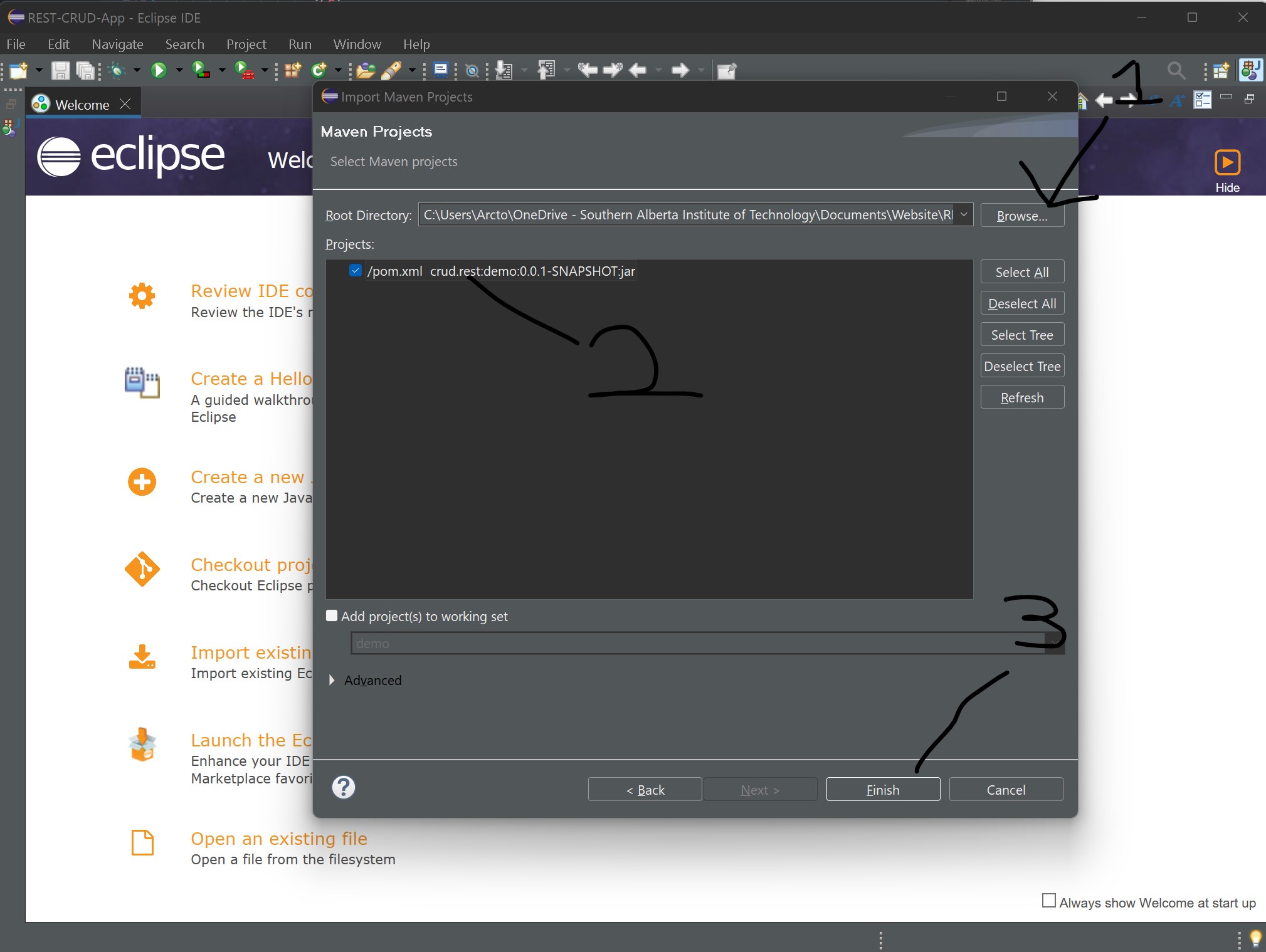
Wait till the project has finished importing and you have successfully imported the project.
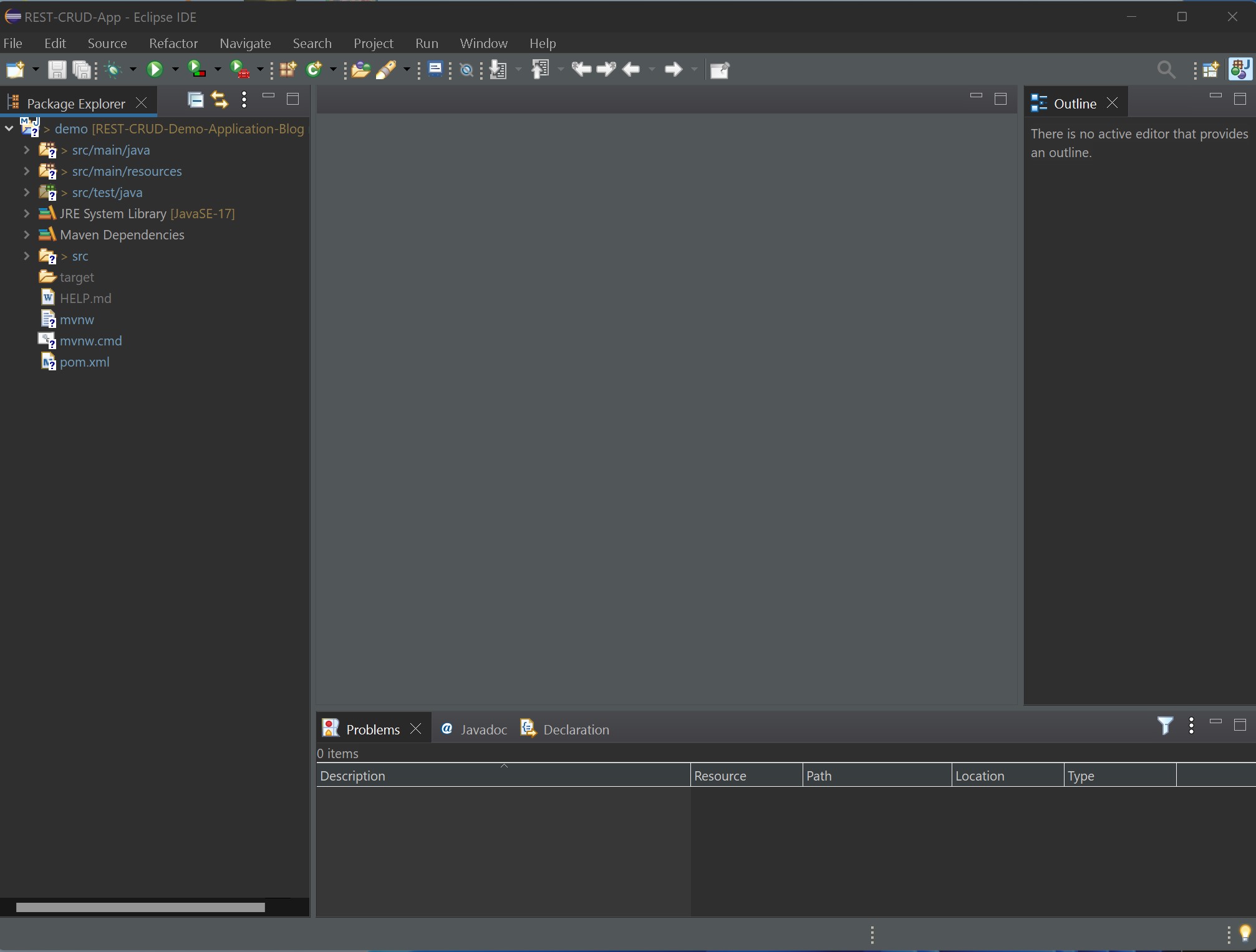
Configurations
We just need to configure the application to link to our database. Click this for the config file which needs to be saved under src/main/resources (there is a file already there, replace this).
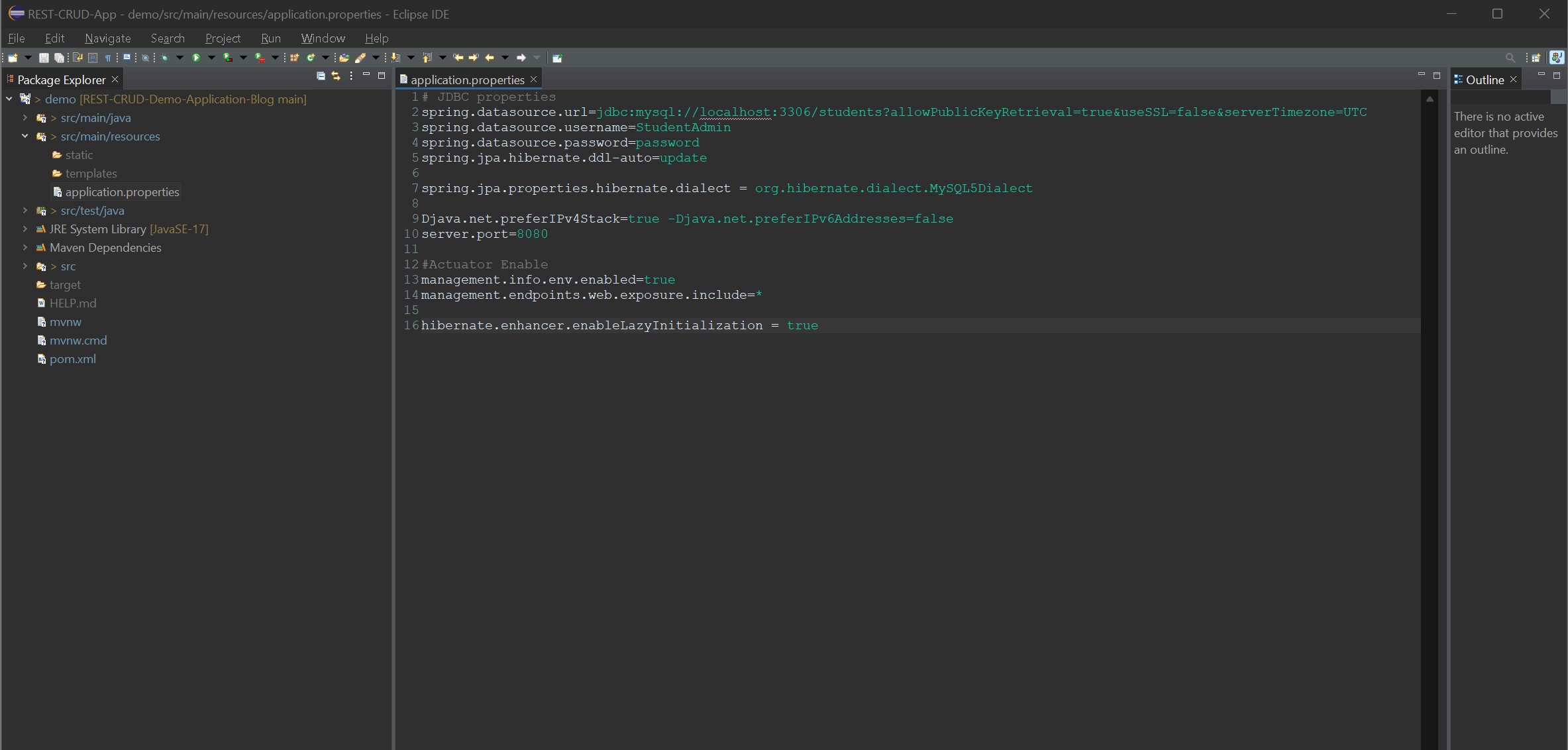
The JDBC properties are the only important part here. They are just to connect hibernate (database java) to MySQL.
- url = Url to connect to database.
- username = Username to connect to DB.
- password = Password to connect to DB.
- dialect = MySQL5Dialect to tell hibernate that the DB is MySQL and that this is the language to interact with the application.
- port = Normally set to 8080 but you can set it to any port number you have available.
Creating the application
This section is about programming the application. I will keep things short and explain the important details as we go along.
Entity/POJO
First we will create the entities for the application. Student and Degree will be our entities.
We will create a package called entity (crud.rest.demo.entity). This package must be under this domain for the Spring Boot application to scan the annotations automatically.
Then we will create two entities under that package, Student and Degree. Let's start with coding the Student Entity.
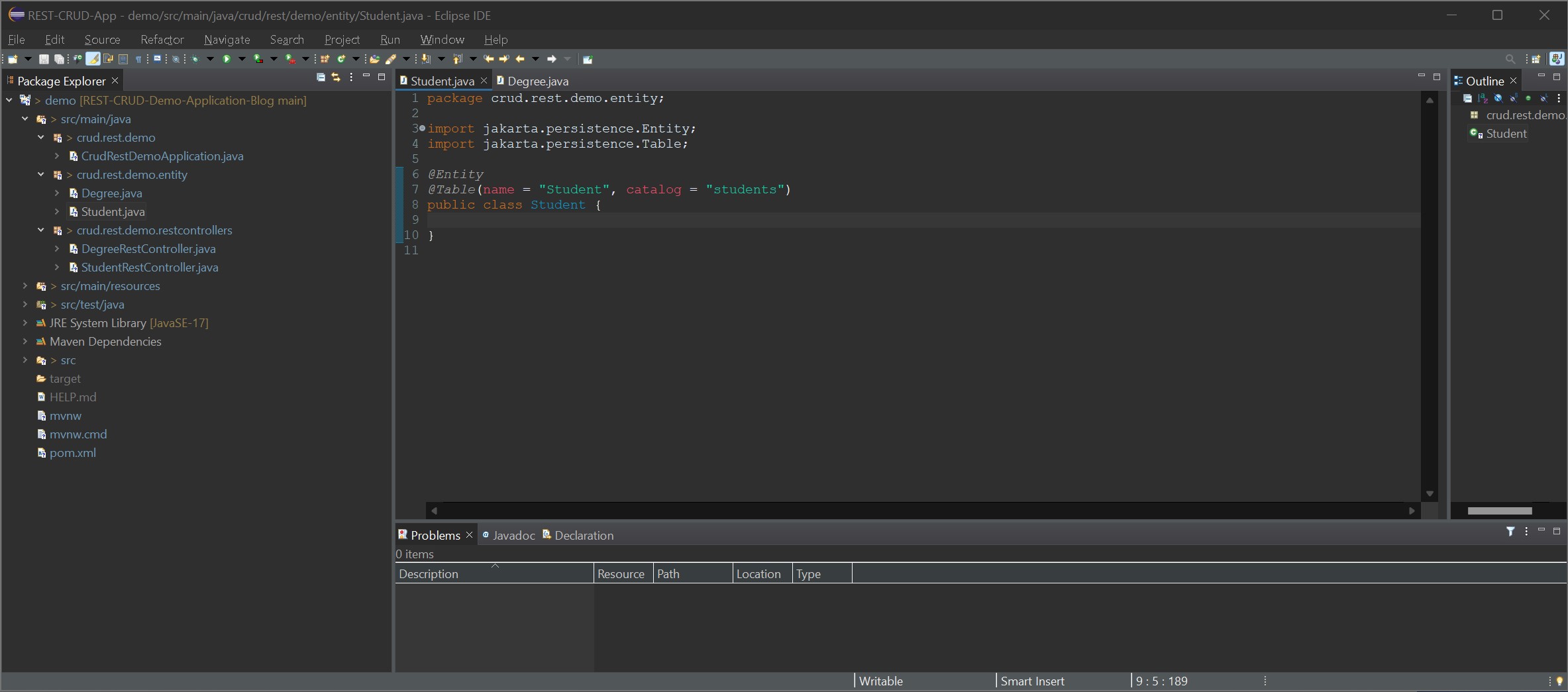
We create the class as we would normally create an object inside java but we add the two annotations.
The first annotation @Entity informs Spring that this class is an entity.
The second anotation @Table informs hibernate that this entity is attached to the student table under the schema callled "students".
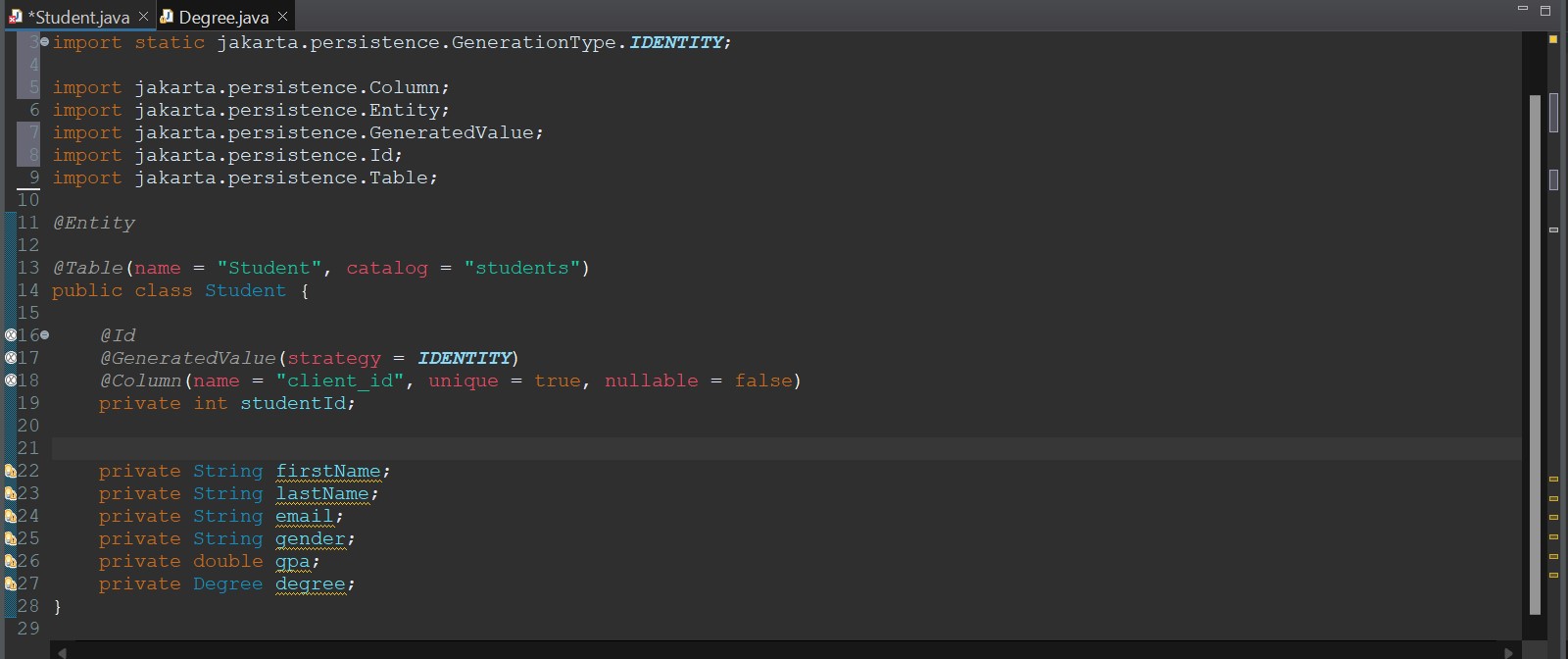
Next we start by creating the fields linked to that table. The id field will have three annotations.
- @ID = Informs hibernate that this is an ID field
- @GeneratedValue = Informs hibernate that the value will be auto-generated
- @Column = Links this field to the column on that table
We will then continue to only add the @Column to the rest of the field until we get to the Degree field.
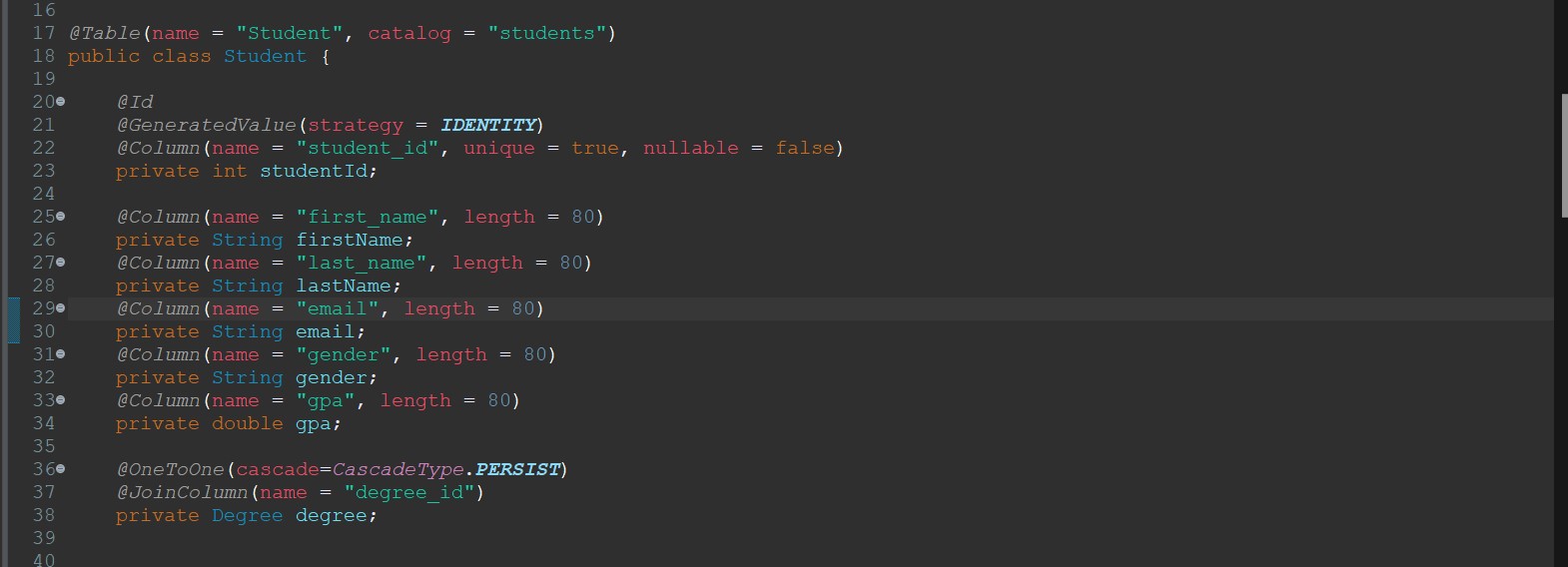
For the Degree field we need to add two new notations
- @OneToOne = Inform hibernate that there is a One To One relationship with this object/table
- @JoinColumn = Links the Student table to the Degree table with the column stated
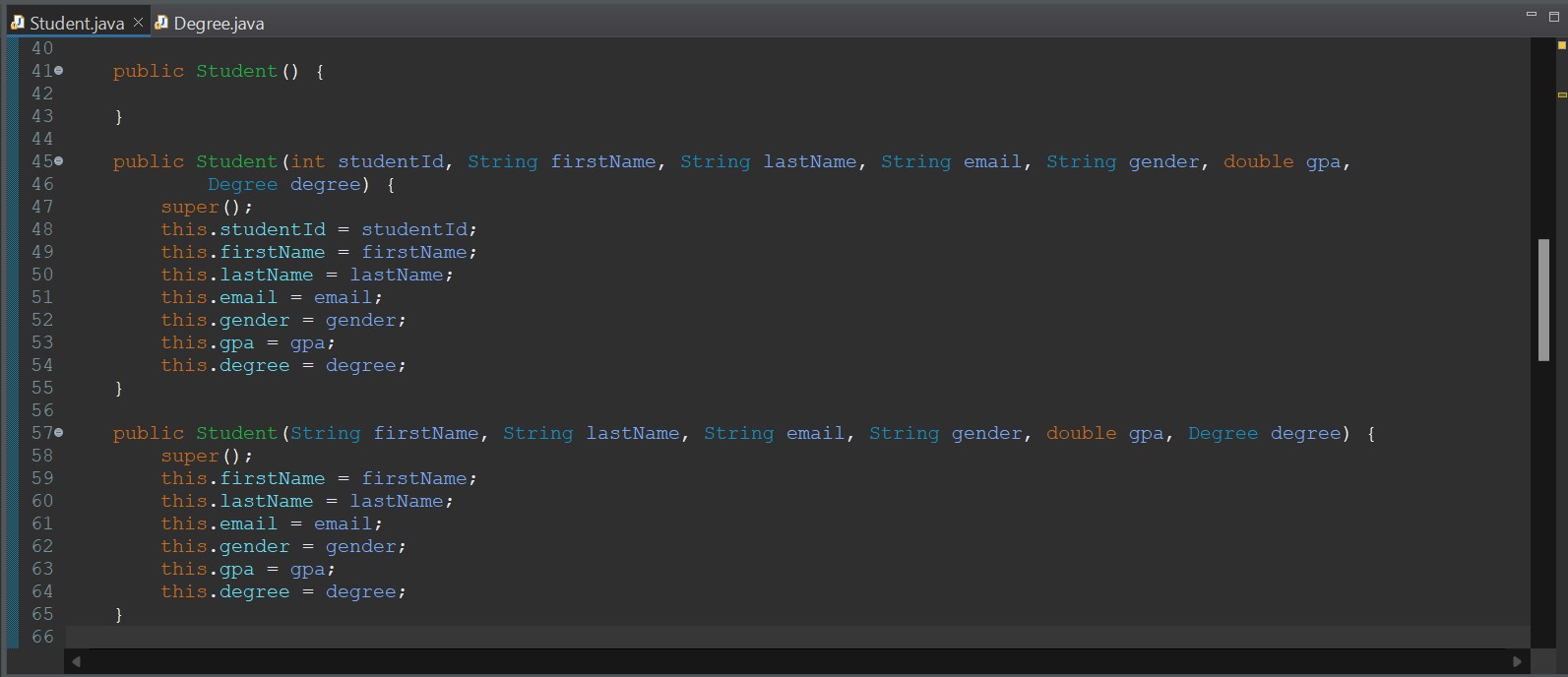
Next we create three constructors, one empty, one with all fields and one without the ID. This is so that hibernate can create the object easily.
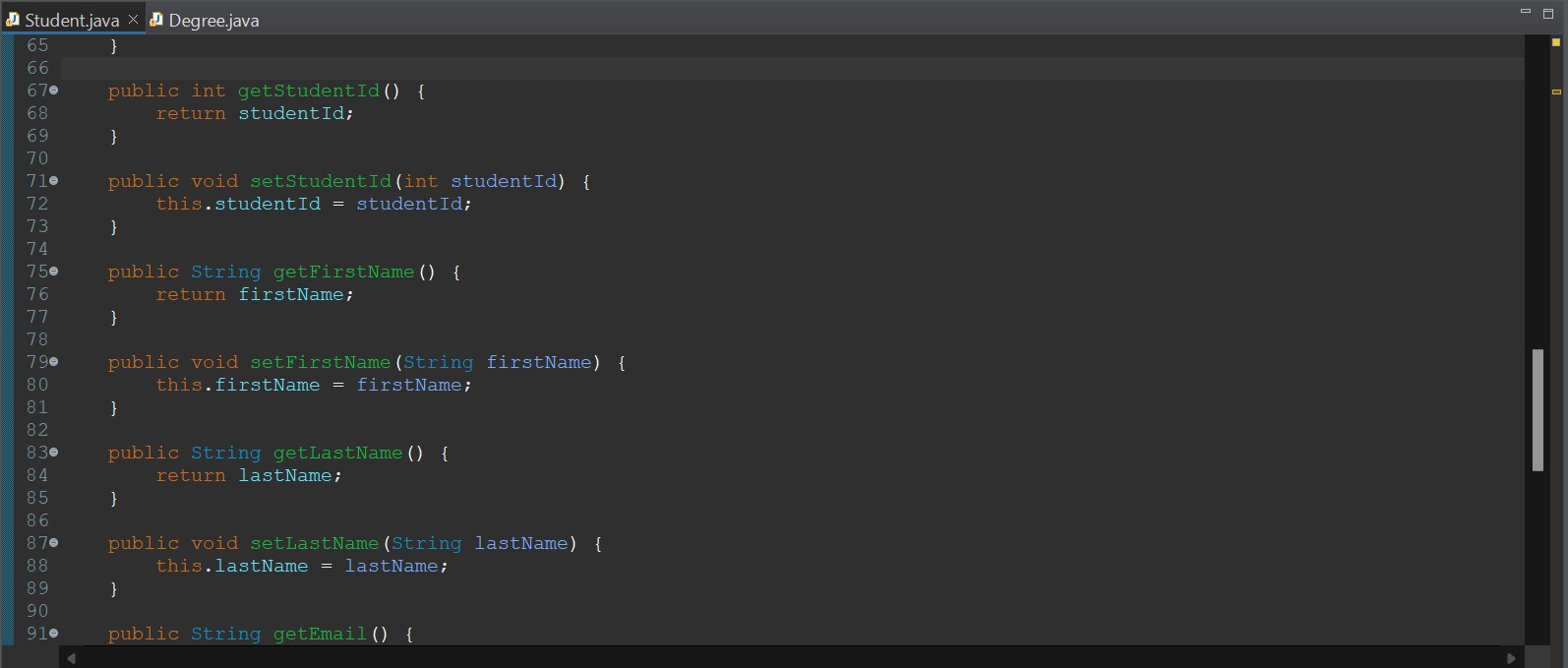
Lastly, we create normal getters and setters for the Student class.
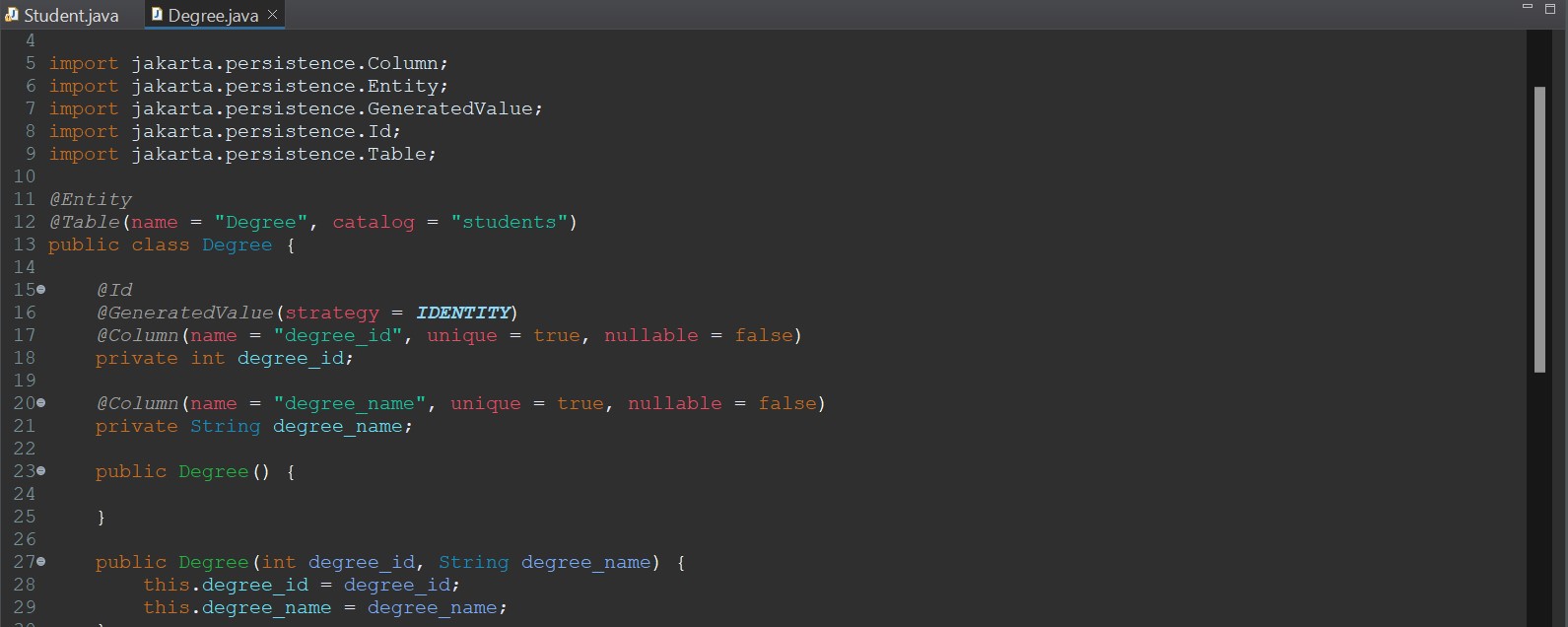
Finally, we do this all again but for the Degree class. Nothing new here.
Github Link for the entities.
Persistence (Hibernate)
The next thing we need to do is to create our persistence classes to enable CRUD (Create, Read, Update, Delete) on the database.
First, we create a new package called DAO (Data Access Object), crud.rest.demo.DAO. Then we create an interface class for the StudentDAO class.
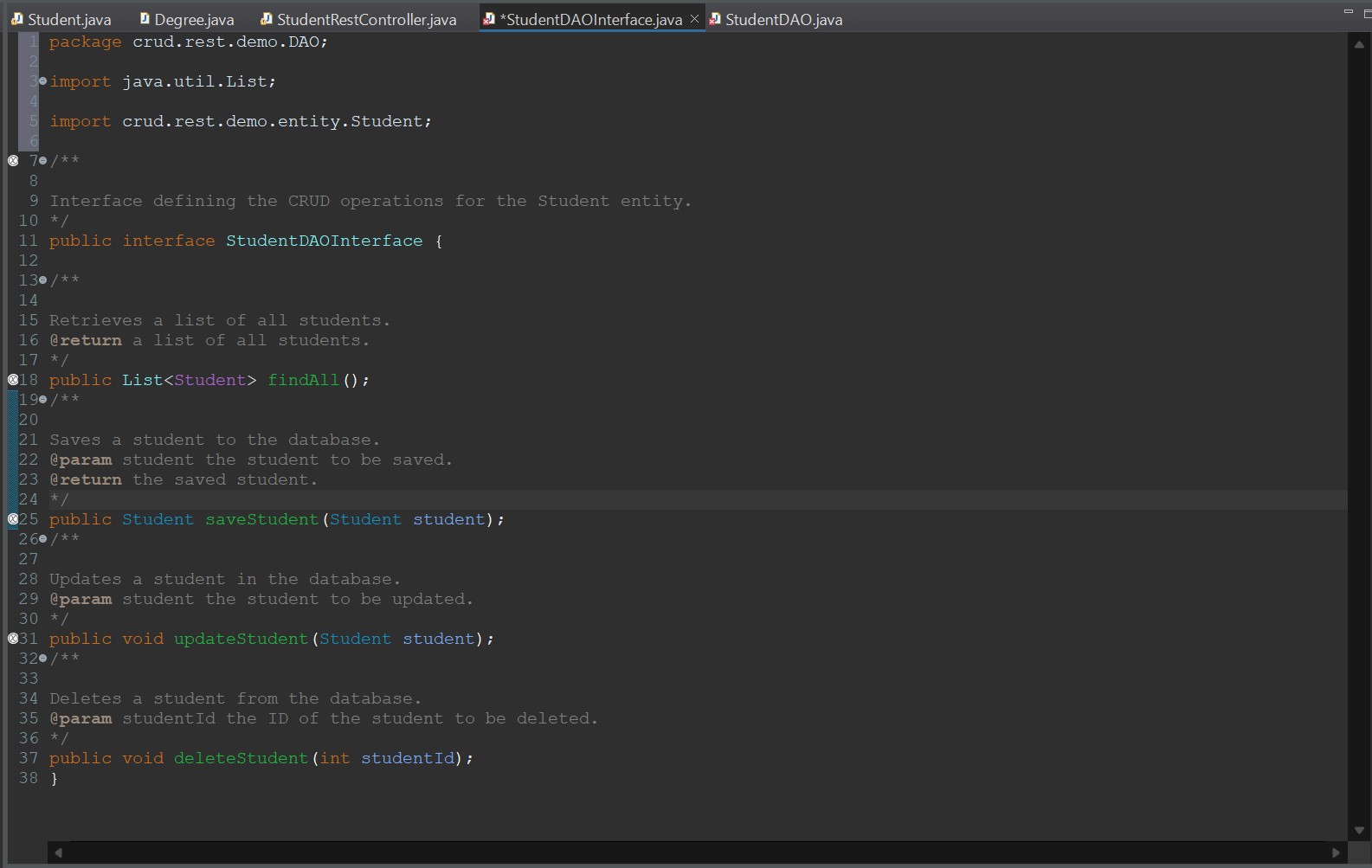
The interface just has the four methods we will use for the CRUD operations.
- findAll - Find all students in the database
- saveStudent - Save a new student into the database
- updateStudent - Update a student in the database with the new details
- deleteStudent - Delete a student from the database
Next, we will create the DAO class and implement these methods.
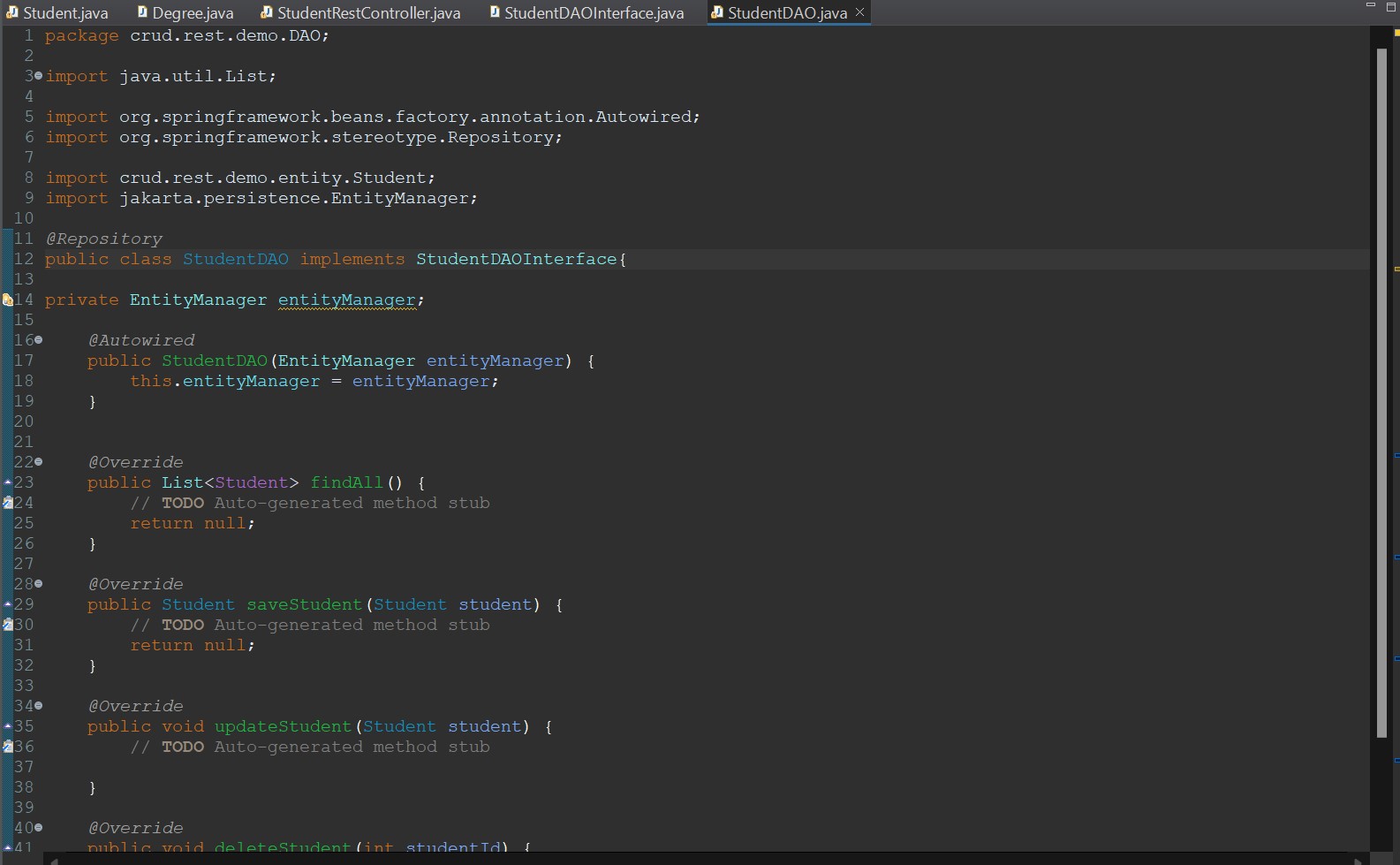
We start by creating the class, implementing the interface, and creating a field. The EntityManager object is what connects to the database and has pre-built methods for us to use within our methods to perform the CRUD operations.
We use the annotation @Autowired to tell Spring to create this object, using this constructor when the application initalizes.
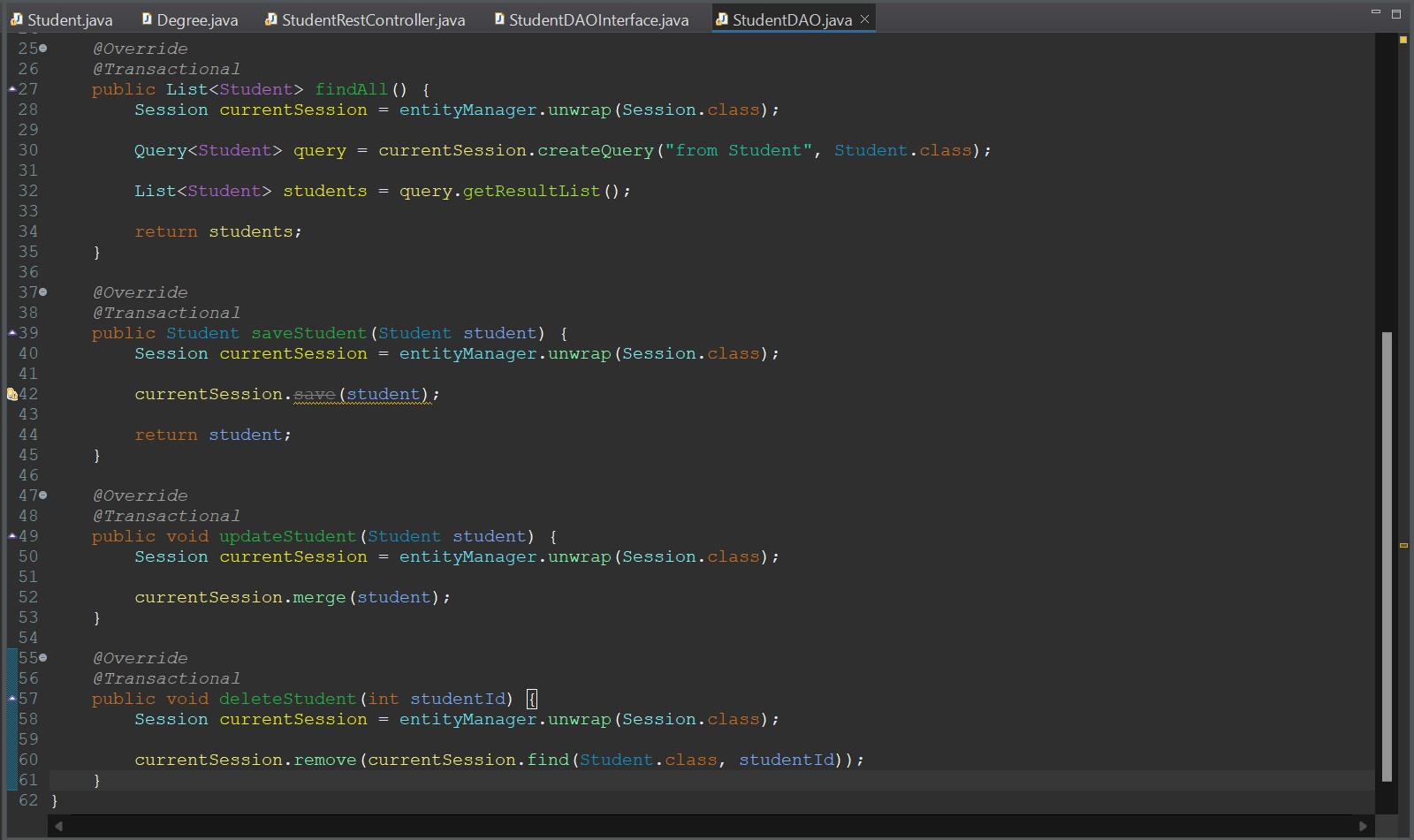
Then inside each method we perform the operations using the entityManager object. We will use the operations below to create the methods.
- @Transactional = Creates a single transaction for this method.
- currentSession.createQuery = Create a query to perform on the database.
- currentSession.save = Saves the object to the database.
- currentSession.merge = Merges the object with the existing object in the database.
- currentSession.find = Creates an object from the table with this ID.
- currentSession.delete = Delete the record from the table inside the database from the object passed.
Now that all these methods are created, we can move on to the final part of the application.
Rest Controller
Next we are going to create the REST Controller for this application. The controller is used to map the endpoints with the HTTP requests and control the data coming in and out.
Let's start with the boilerplate for the Student Rest Controller Class.
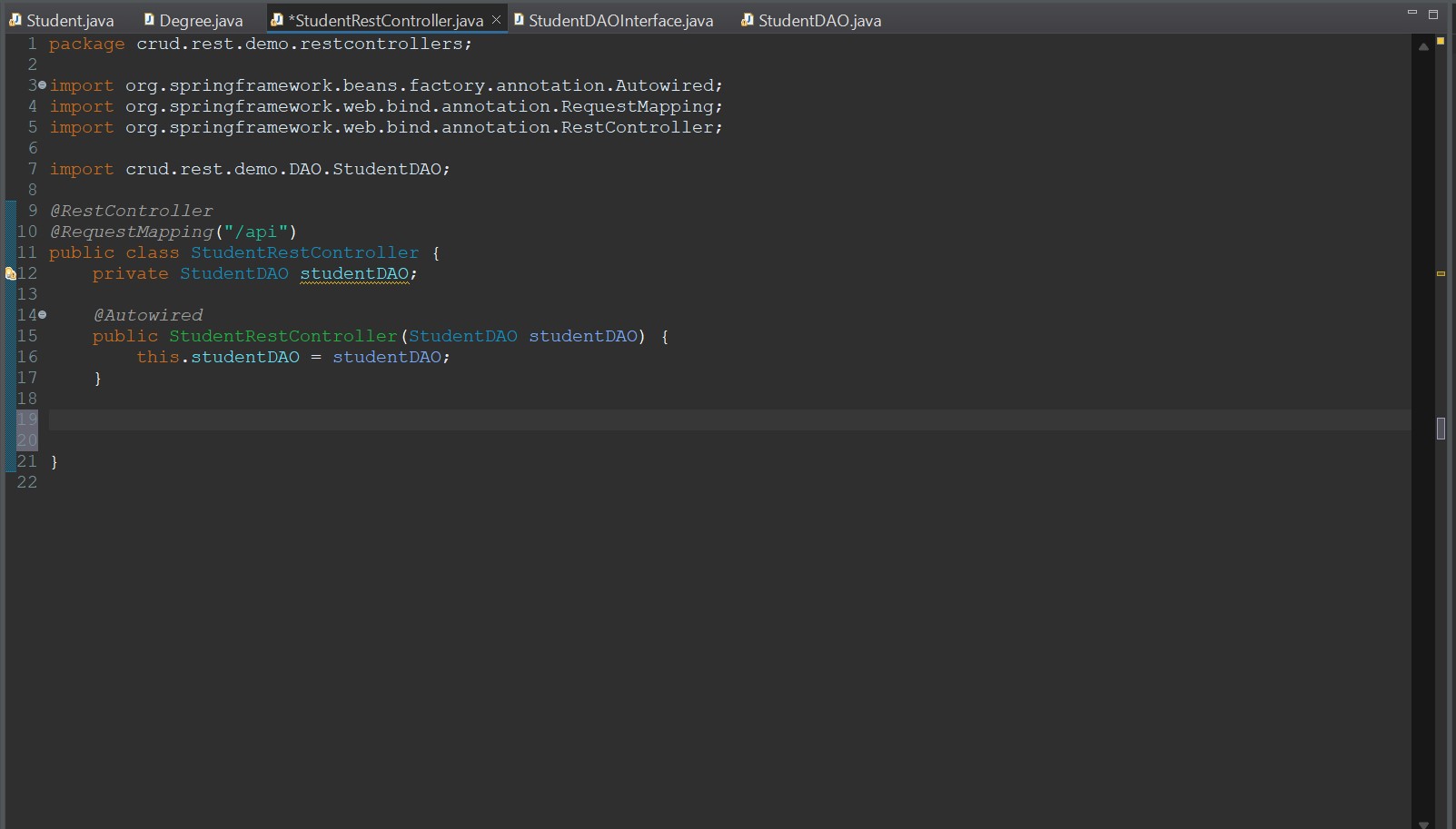
We create the class and initialize the class with the studentDAO object in order to perform the operations.
- @RestController - Informs Spring that this is a Rest Controller class.
- @RequestMapping - Create an endpoint at the string passed (localhost:8080/api).
Next we fill out the methods with the endpoints and HTTP requests.
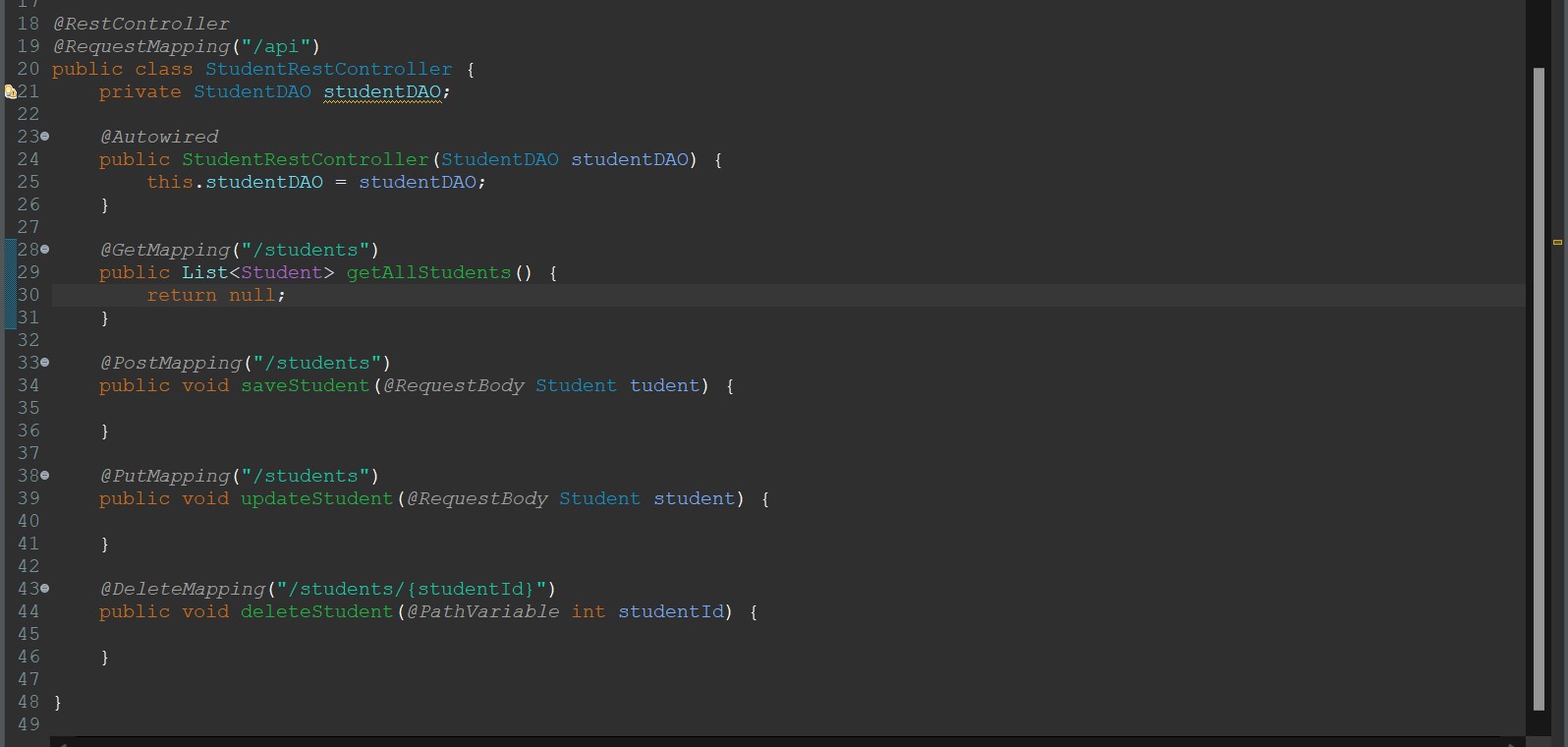
- @GetMapping - Creates a GET endpoint at localhost:8080/api/students, which will be used to obtain the students from the database.
- @PostMapping - Creates a POST endpoint at localhost:8080/api/students, which will be used to save a new student in the database.
- @PutMapping - Creates a PUT endpoint at localhost:8080/api/students, which will be used to update a student in the database.
- @DeleteMapping - Creates a DELETE endpoint at localhost:8080/api/students/{studentId}, which will be used to delete a student from the database with the student id (replace the {studentId with the id you want to replace}).
- @RequestBody - Obtains the JSON inside the body of the request and creates a student object (Jackson automatically converts the JSON for us).
- @PathVariable - Converts the {studentId} into an int for the method to use.
Now we just need to finish writing the methods by using the StudentDAO class to perform the CRUD operations for us.
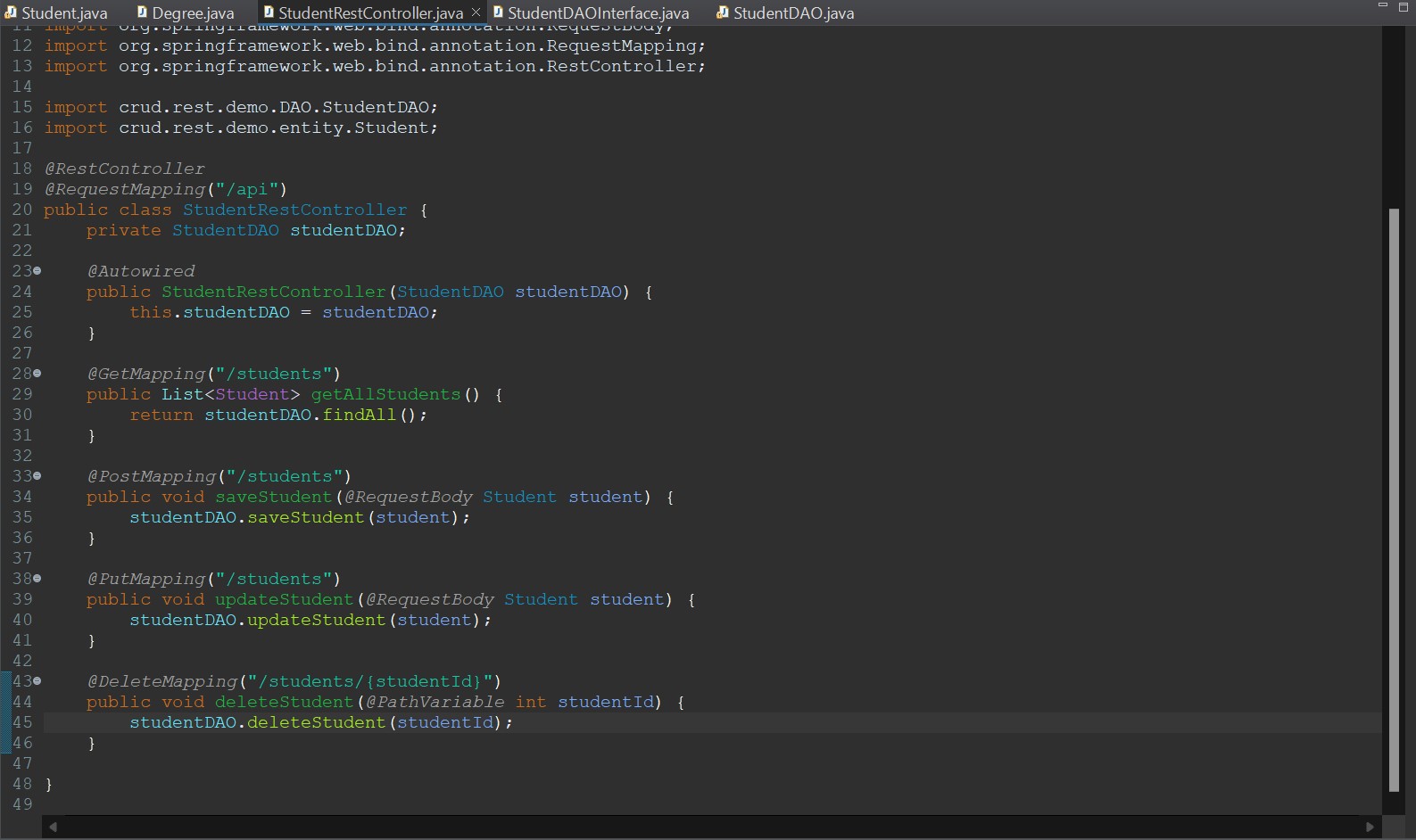
Now the application has been completed. Github Link for the REST application section.
Testing
Now we will test each endpoint to ensure that they are working. , the Postman application. You can download it here.
Postman allows us to perform HTTP requests, which we will use to test our REST application.
GET Request Test
We will perform a GET request at the URL localhost:8080/api/students
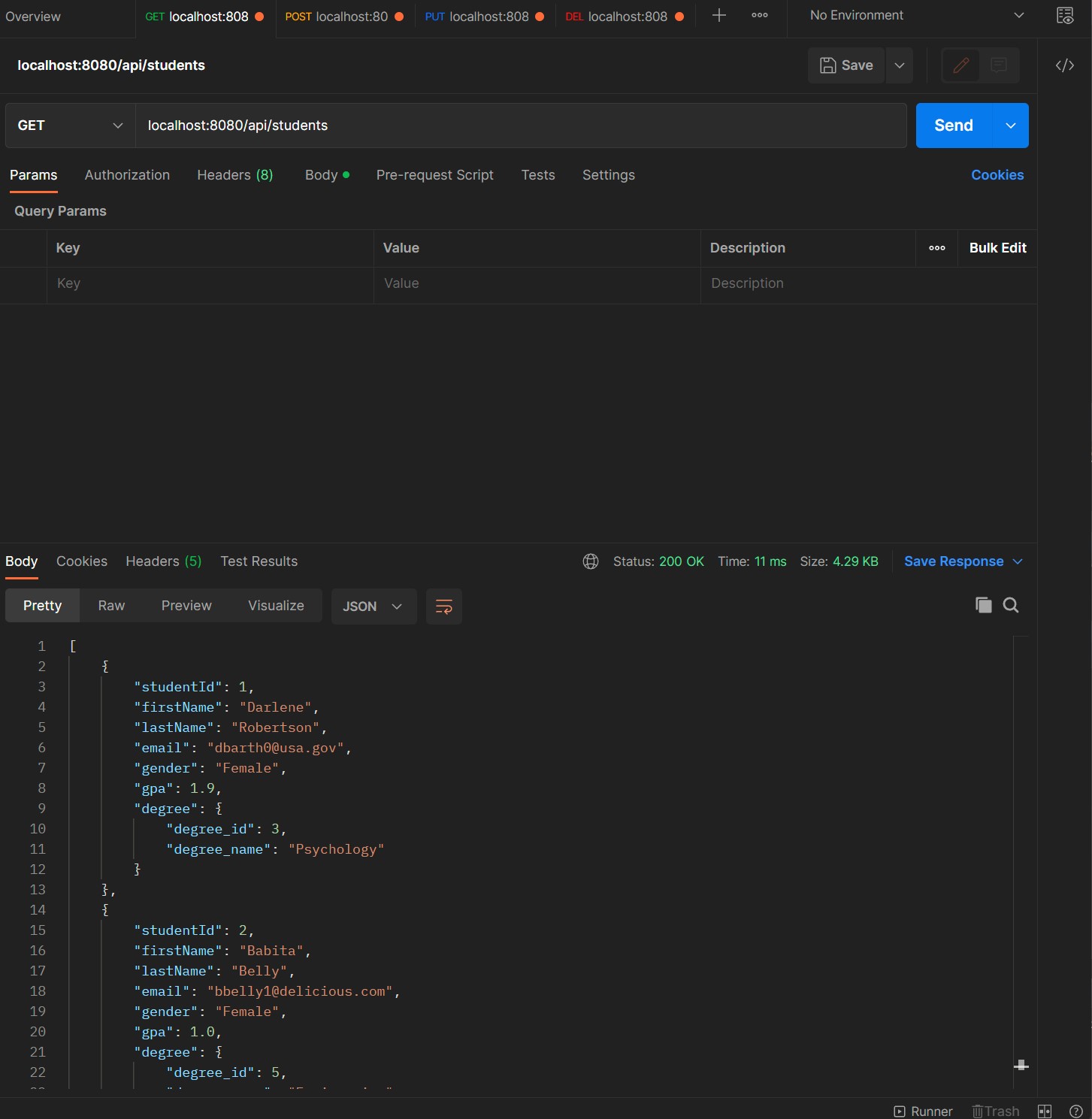
At the bottom, we can see that all the students have been converted into JSON for us, which was obtained from the database.
POST Request Test
We will perform a POST request at the URL localhost:8080/api/students
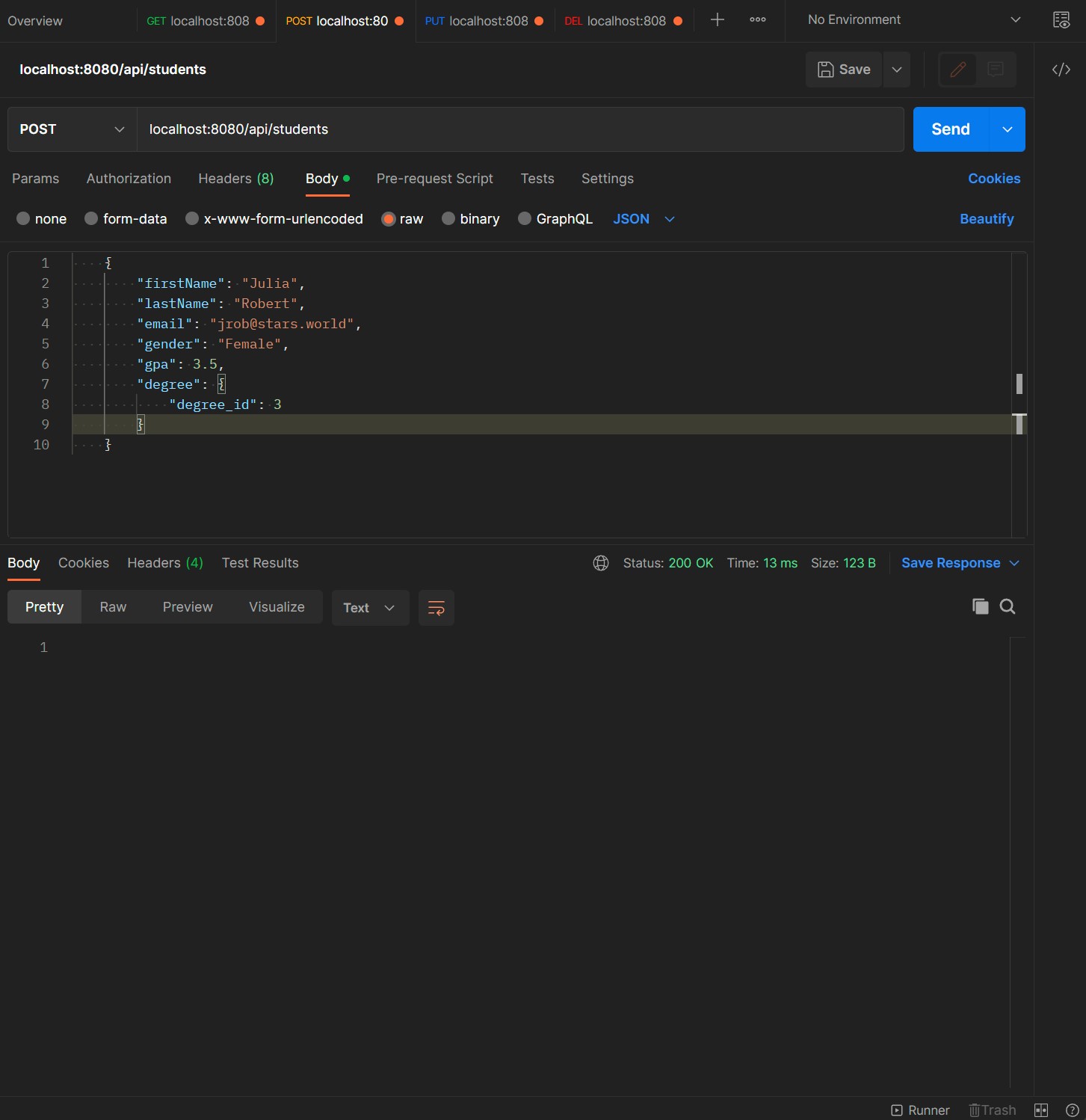
For this, we need to set the JSON object inside the body of the reqeust as RAW/JSON data.
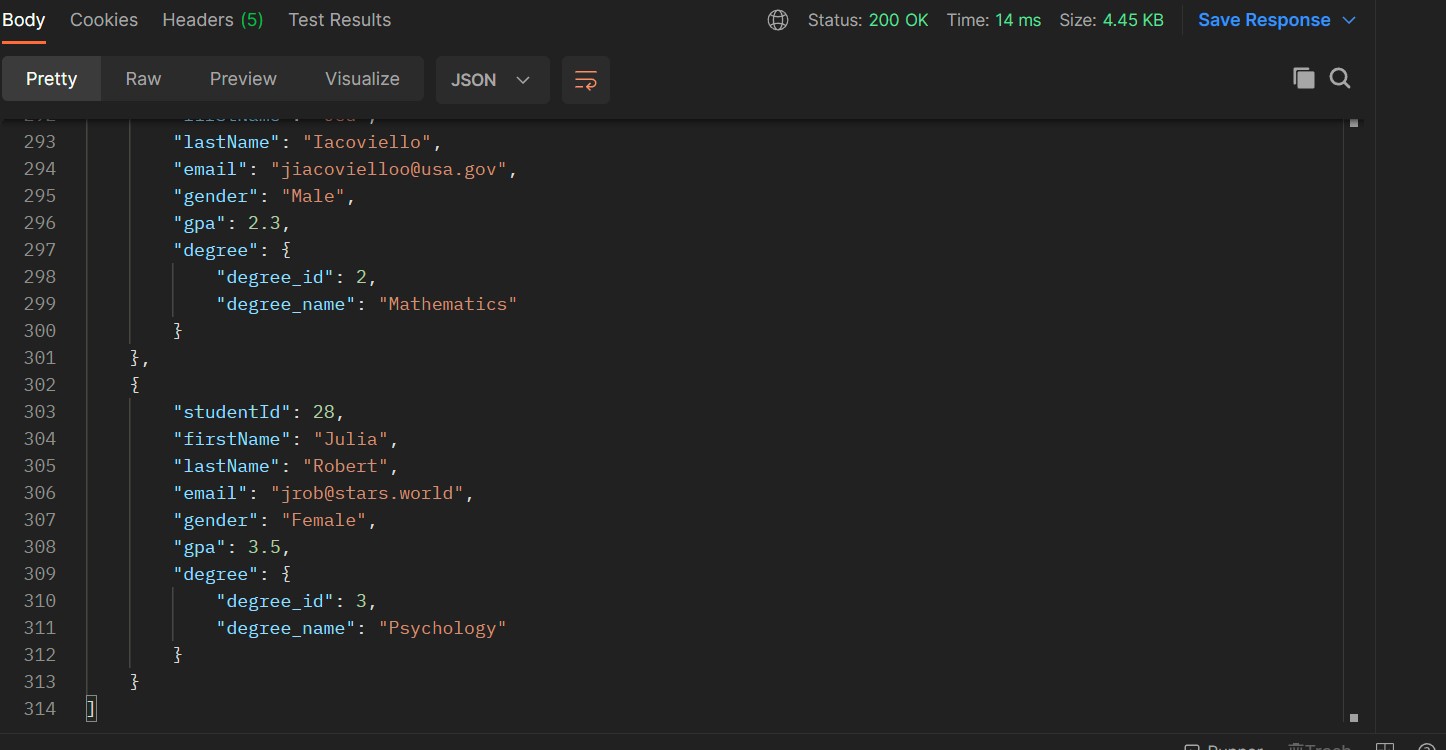
As you can see the data was now saved into the database.
PUT Request Test
We will perform a PUT request at the URL localhost:8080/api/students
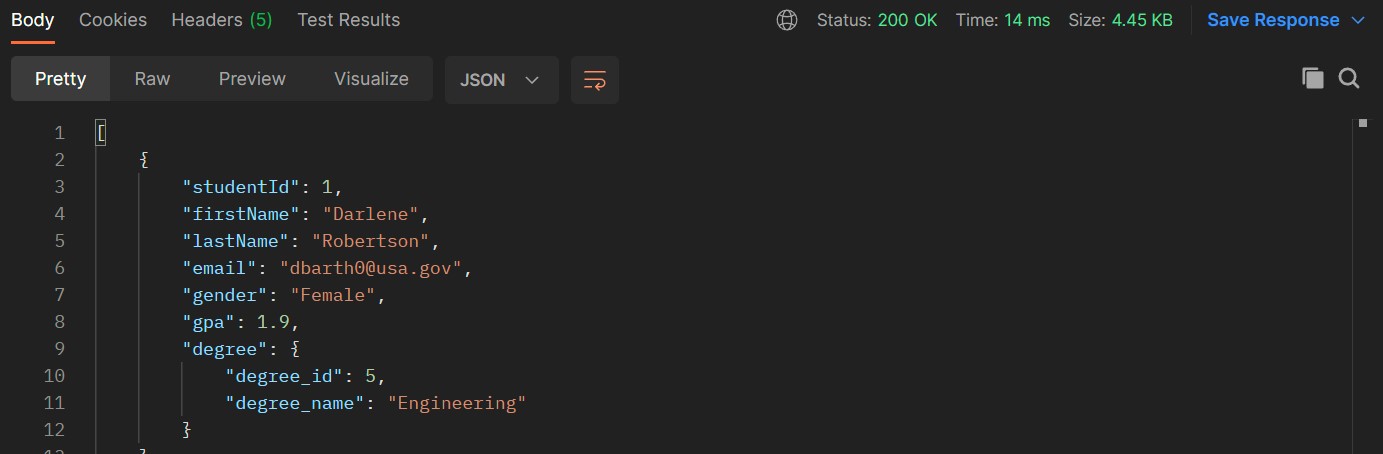
We will update the student with the studentId 1.
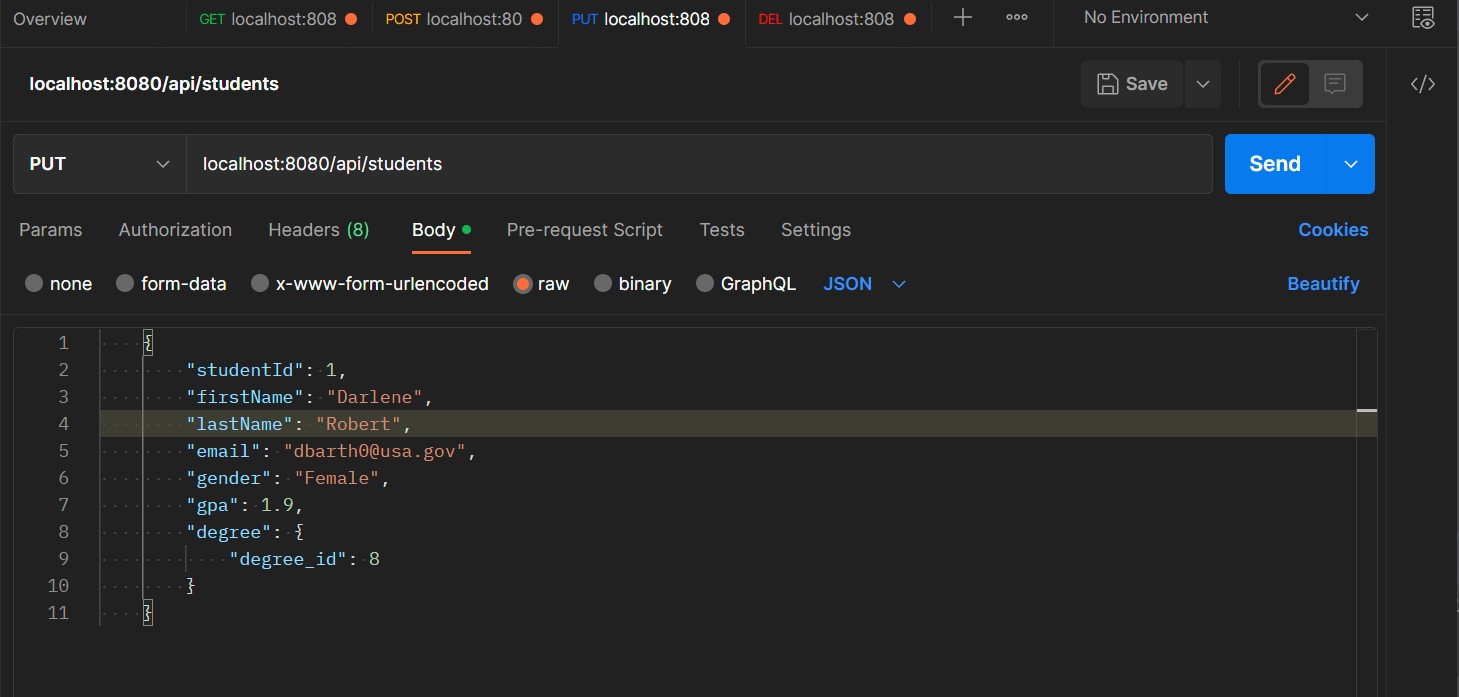
Lets change her last name to Robert and her degree to id 8.
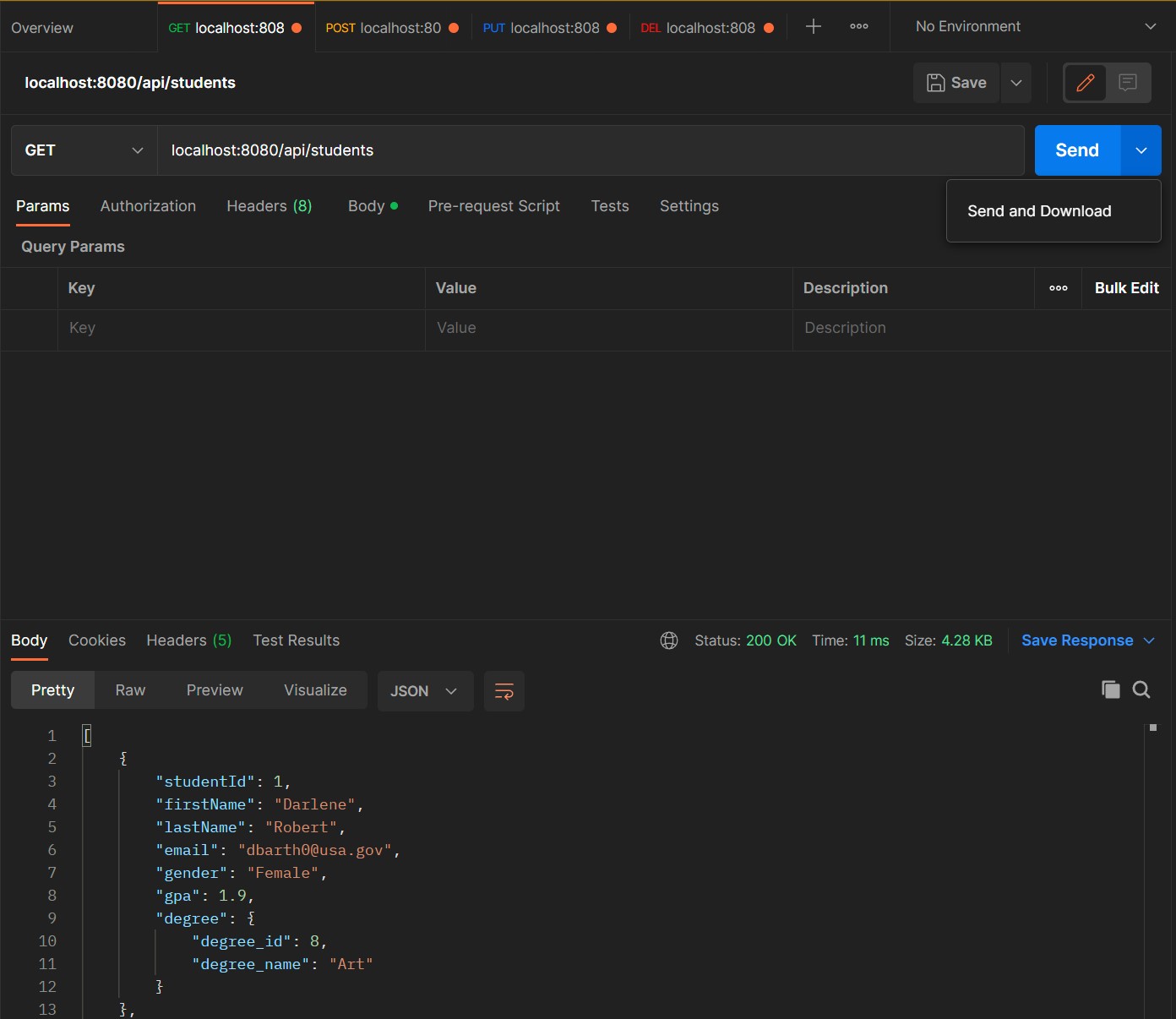
As you can see here the last name has changed to Robert and she is now taking Art as her degree.
DELETE Request Test
We will perform a DELETE request at the URL localhost:8080/api/students/28
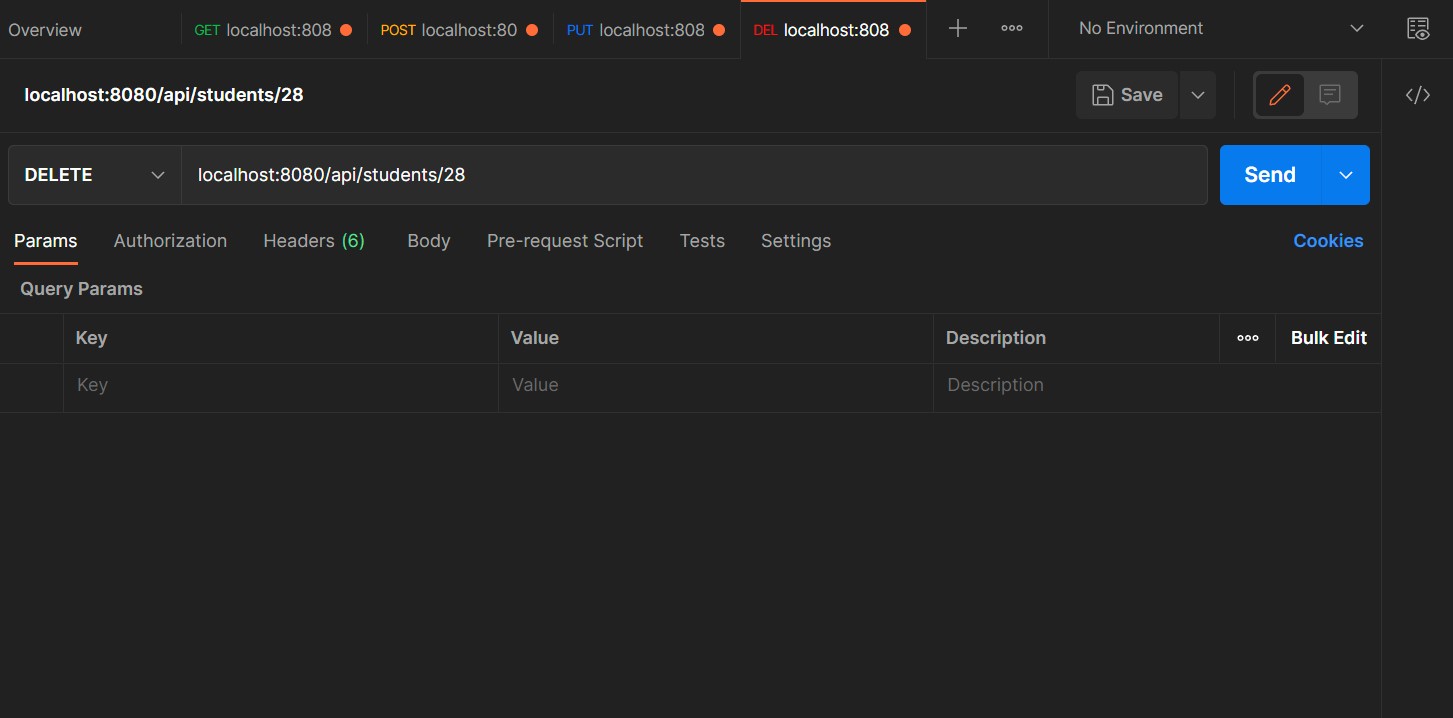
We will delete the student with the studentId 28.

As you can see there is no student in the database with ID 28 anymore.
Success, all the test worked and the application is fully up and running. Wooo!
More Info
Thank you for reading my guide to setting up a simple REST application.
The GitHub code for the project can be found here.
If you would like to learn more about Spring and Spring Boot, please check out this course of Udemy.